Collections- Need of Collection Framework
I want to represent one value by one variable , 2 values with 2 variable etc , we will have no problem .
int y=20;
int z=30;
Now if we want to represent huge values, declaring 10,000 variable is worst type of programming practice . Readability of the code is going to get down.
To overcome this problem we should go for next level that is Arrays.
Normally, array is a collection of similar type of elements that have contiguous memory location.
------------------------------------------------------------------------------------------------------------
Overview of Array:-
+Java array is an object the contains elements of similar data type. It is a data structure where we store similar elements. We can store only fixed set of elements in a java array.
Array in java is index based, first element of the array is stored at 0 index.
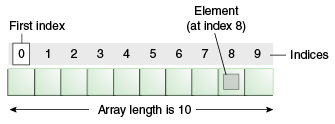
- int a[]=new int[5];//declaration and instantiation
- a[0]=10;//initialization
- a[1]=20;
- a[2]=70;
- a[3]=40;
- a[4]=50;
Syntax with values given (variable/field initialization):
int[] num = {1,2,3,4,5};
Multidimensional array
Declaration
int[][] num = new int[5][2];
Initialization
num[0][0]=1;
num[0][1]=2;
num[1][0]=1;
num[1][1]=2;
num[2][0]=1;
num[2][1]=2;
num[3][0]=1;
num[3][1]=2;
num[4][0]=1;
num[4][1]=2;
Or
int[][] num={ {1,2}, {1,2}, {1,2}, {1,2}, {1,2} }
Advantage of Java Array
- Code Optimization: It makes the code optimized, we can retrieve or sort the data easily.
- Random access: We can get any data located at any index position.
Disadvantage of Java Array
- Size Limit: We can store only fixed size of elements in the array. It doesn't grow its size at run time. To solve this problem, collection framework is used in java.
------------------------------------------------------------------------------------------------------------
Arrays :
Student[] s=new Student [100000];
Biggest advantage of array is we can represent huge number of values by using a single variable , so that readability of the code is going to be improved.
Problem with arrays is that :
1. Arrays are fixed in size , once we declare an array size of the array will be fixed .We cannot increase or decrease size of an array.
Suppose i have started java training and i have got 10,000 applicants who are interested in learning java . I have arranged 10,000 chairs for each student to sit.
At run time only 200 students came . What will happen to the remaining chairs , it will become waste.
Now based on the previous experience i started a new batch and i though around 200 students is gonna come . So i have arranged only 200 chairs . Now 10,000 students came at run time. Will i be in a position to provide support to remaining students . Answer will be "NO".
Same case happens for Arrays too.
So for arrays concept compulsory we have to know the size in advance which may or may not be possible at run time.
2. Arrays can hold only homogeneous data elements . Suppose we have created a student array:
Student [] s = new Student[10000];
Here we can represent only student type of information .
s[0]= new Student(); ---valid
s[1]= new Customer();----invalid
compile time error : expecting student type object but will get customer type object . So incompatible type error we are going to get.
Incompatible type found : required student but found Customer.
So what will be the solution to this problem :
We can solve this problem by using object type array.
Object []a= new Object[10000];
a[0]= new Student ();----valid
a[1]= new Customer();----valid
Because student and customer are of object type , so happily we can go for Object type arrays as both Student and Customer are of type Object.
3. Arrays concept is not implemented based on some standard data structure , but every collection class is implemented based on some standard data structure. That is why underlying data structure or ready made method support we can't expect from arrays.
For every requirement we have to compulsory write the code.
Suppose i want to inset elements into an array and all elements must be inserted in some sorting order , then who is responsible to write the sorting logic ?
The programmer is responsible to write.
Check if an element is there or not : We do not have ready made method support for it.
So it will be the programmer responsibility to write down the sorting logic for it.
So ready made method support is not available for each and every requirement . Compulsory we have to write down the code.
If we want to enter elements based on some sorting order , better to go for tree set .
If we want to search a particular element is there or not , collection contains a method called contains .
So for every requirement ready made method support , you can expect in collection but in Arrays you can't.
So we can say that collections concept provide solutions for all limitations of an Array.
Which concept is recommended to use : Arrays or Collections?
If you know size in advance , highly recommended to go for Arrays concept. Collections are growable in nature that is based on our requirement we can increase or decrease the size. This growable nature we are not going to get free of cost . What are are paying or convincing is : PERFORMANCE.
example :
Suppose we have an array of size 10.
If 11th element comes , array will not be able to provide support.
Assume ArrayList:
ArrayList of 10 element :
If 11th element comes , ArrayList will not be in a position to provide support.Do not feel that cell is going to get automatically created and 11th element is going to get stored.
What happens internally is once ArrayList reaches its max capacity a bigger array list object will be created internally.
ArrayList uses an array to store elements . Arrays have a fixed size . The array that arrayList uses has to have a default size, obviously. 10 is a probably a more or less an arbitrary number for the default number of elements.
When you create a new ArrayList with nothing in it , then ArrayList will have made an array of 10 elements behind the scenes . Of course those 10 elements are all null.
Each time the array is full, ArrayList creates a new larger array and copies the elements from the old array to the new array. You don't want this to happen every time you add an element to the ArrayList.
Copying the array takes time especially if the array size is larger .So array list does it in the steps of 10 or so.
(If you want to know how it works , look for the files src.zip in your JDK instala\lation directory .pen it and look up the sorce code for java.util ArrayList).
1 comment:
Thanks for your information. very good article.
Best Selenium Course Online
Selenium with Java Online Training
Post a Comment